C++ - "shared_ptr"的使用方法 及 代码
"shared_ptr"的使用方法 及 代码智能指针(smart pointer)是C++11的新特性. 指针在无人使用时, 自动释放动态内存.
通过"use_count"计数, 并判断是否无人使用, 从而释放内存.
在函数复制(copy)过程中, 默认copy方法是引用相同的潜在元素.
如下代码, b1和b2共享元素, 如果范围结束({}), 则b2释放, b1则没有元素, 为了保持b1中的元素, 而使用动态内存.
由于VS2012很多C++11特性无法支持, 所以在Eclipse CDT上进行测试(GCC4.8.1).
代码(Eclipse CDT)如下, 注意b1的元素个数, 以及shared_pointer的使用数(use_count):
/* * CppPrimer.cpp * * Created on: 2013.11.2 * Author: C.L.Wang */ /*eclipse cdt*/ #include <iostream> #include <vector> #include <string> #include <initializer_list> #include <memory> #include <stdexcept> using namespace std; class StrBlob { public: typedef std::vector<std::string>::size_type size_type; StrBlob(); StrBlob(std::initializer_list<std::string> il); size_type size() const{ return data->size(); } bool empty() const { return data->empty(); } void push_back(const std::string& t) { data->push_back(t); } void pop_back(); std::string& front() ; std::string& back() ; std::shared_ptr<std::vector<std::string> > data; //test private: //std::shared_ptr<std::vector<std::string> > data; void check(size_type i, const std::string &msg) const; }; StrBlob::StrBlob():data(std::make_shared<std::vector<std::string>>()) {} StrBlob::StrBlob(std::initializer_list<std::string> il): data(std::make_shared< std::vector<std::string> >(il)) {} void StrBlob::check(size_type i, const std::string &msg) const { if(i >= data->size()) throw out_of_range(msg); } std::string& StrBlob::front () { check(0, "front on empty StrBlob"); return data->front(); } std::string& StrBlob::back () { check(0, "back on empty StrBlob"); return data->back(); } void StrBlob::pop_back () { check(0, "pop_back on empty StrBlob"); data->pop_back(); } int main (void) { StrBlob b1; //{}释放b2 { StrBlob b2 = {"a", "an", "the"}; b1 = b2; b2.push_back("about"); } std::cout << "b1 size = " << b1.size() << std::endl; std::cout << "b1 data use count = " << b1.data.use_count() << std::endl; //b2已经被释放了 //std::cout << "b2 size = " << b2.size() << std::endl; return 0; }
输出:
b1 size = 4
b1 data use count = 2
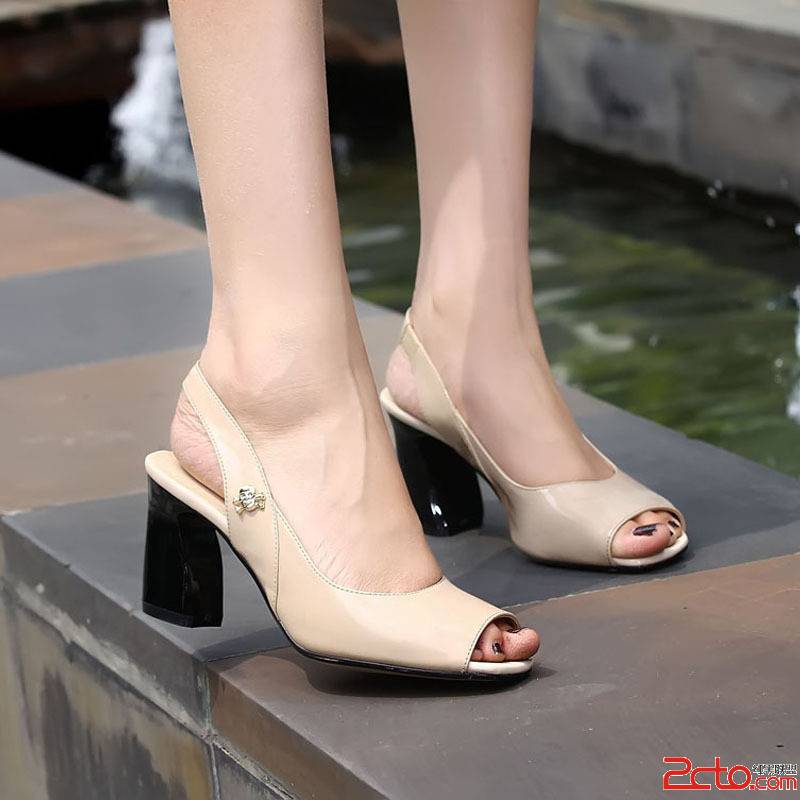
补充:软件开发 , C++ ,